AutoViewModel
AutoViewModel is a Fluent MVVM features to auto instantiate our
ViewModels
classes. Its objective is simplified as far as possible ViewModels
classes with same performance and stability.
AutoViewModelmark> isn’t mandatory, so that we can use any other technique.
AutoViewModelmark> has a basic rule that showing the following image.
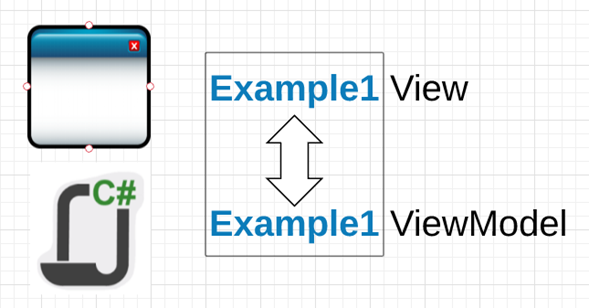
The
View
class name and the ViewModel
class name have to be the same preceded by suffix View for the View
class and ViewModel for the ViewModel
class.
- SameNameClass[View].xaml / cs
- SameNameClass[ViewModel].cs
A Example
- MyView.xaml / cs
- MyViewModel.cs
ViewModel
class following the general autoInstace rule so that, its name will be MyViewModel.cs.
AutoViewModel Class
AutoViewModel.cs
class is a Fluent MVVM ViewModel
instance control class. It is in MoralesLarios.FluentMVVM.Infrastructure
namespace and is a static class with 2 AttachDependency
properties.
- IsAutoMaticViewModelInstance
- Is a
bool
property,false
is its default value. If this property istrue
, AutoViewModel engine will try to instantiate its ViewModel class referred (this class must comply with the previous main nomenclature rule). If isfalse
, it will do nothing. - ViewModelClassName
- Is a
string
property. If your ViewModelClass doesn’t follow the main nomenclature rule, you set the ViewModelClass name in this property.
Internal Class Search Rule
When AutoViewModel engine search ViewModelClass to instantiate, follow the next rule:
In the case we have added an
In the case we have added an
IoC
in our project, this will be the first place to search. If there isn’t IoC
or ViewModelClas isn’t in the IoC
configurations types, The AutoViewModel engine search in all project. This rule is valid to two different searches (IsAutoMaticViewModelInstance
and ViewModelClassName).
Important: If you don’t setup your
ViewModel
class in IoC
, your ViewModel
class a constructor without parameters
.
Examples
This are 4 types
Our example has an initial Fluent MVVM configuration only with
We will build 2
Will change
We show
AutoViewModel
configuration. We will create an example for each.
Our example has an initial Fluent MVVM configuration only with
nuget package
and IoC
configuration. For more info QuickStart and Multi IoC sections.
We will build 2
Views
and 2 ViewModels
, a couple for those who comply with nomemclature rules and another for those who don’t comply.
Will change
IoC
configuration to demonstrate it has preference over another project classes.
We show
IoC
Fluent MVVM configuration with empty instances registered.
using Autofac; using MoralesLarios.FluentMVVM.Infrastructure.IoC; using System; namespace FluentMVVMExamplesDesc.AutoViewModelApp.IoC { class BootStrapper : IContainerIoCWorker { public IContainer Container { get; private set; } public void RegisterTypes() { var builder = new ContainerBuilder(); // Empty Container = builder.Build(); } public object ResolveObject(Type type) { if (type == null) return null; object result = null; result = Container.TryResolve(type, out result); return result; } public T ResolveType<T>() { if (type == null) return null; T result = default(T); Container.TryResolve<T>(out result); return result; } } }
Example 1: ViewModel class with nomenclature rule without IoC
-
The first step is add a simple
WPF Window View
WithRuleView.xaml .
-
The second step is add
ViewModel
WithRuleViewModel.xaml . -
Completed code in WithRuleView.xaml .
<Window x:Class="FluentMVVMExamplesDesc.AutoViewModelApp.WithRuleView" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:local="clr-namespace:FluentMVVMExamplesDesc.AutoViewModelApp" xmlns:fluent="clr-namespace:MoralesLarios.FluentMVVM.Infrastructure;assembly=MoralesLarios.FluentMVVM" fluent:AutoViewModelClass.IsAutomaticViewModelInstance="True" mc:Ignorable="d" Title="WithRuleView" Height="359" Width="602"> <Grid> </Grid> </Window>
AS we can check it meets naming rule.
WithRuleViewModel.cs code.
namespace FluentMVVMExamplesDesc.AutoViewModelApp.ViewModels { using MoralesLarios.FluentMVVM; public class WithRuleViewModel : ViewModelBase { public WithRuleViewModel() { } } }
ViewModel
class automatically.
Result.
When we auto instantiate non IoC setup classes, these classes have to be parameterless constructor, otherwise, they will throw an exception
.

All process in video.
Example 2: ViewModel class with nomenclature rule with IoC
To give more value to example, we will add a dependency injection in our
ViewModel
.
public class WithRuleViewModel : ViewModelBase { private readonly IMyInjection _myInjection; public WithRuleViewModel(IMyInjection myInjection) { _myInjection = myInjection; } }
In this moment will add our
WithRuleViewModel
and MyInjection
classes in IoC
configuration. With this action
public void RegisterTypes() { var builder = new ContainerBuilder(); builder.RegisterType<WithRuleViewModel>().AsSelf(); builder.RegisterType<MyInjection>().As<IMyInjection>(); Container = builder.Build(); }
Result.
All process in video.
Example 3: ViewModel class without nomenclature rule with IoC
It’s time to add a new
View
and a new ViewModel
. For these new examples don’t must not comply the nomenclature rule.
-
The first step is add a simple
WPF Window View
WithoutRuleView.xaml .
-
The second step is add
ViewModel
NoRuleViewModel.xaml . -
Completed code in WithoutRuleView.xaml .
<Window x:Class="FluentMVVMExamplesDesc.AutoViewModelApp.WithRuleView" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:local="clr-namespace:FluentMVVMExamplesDesc.AutoViewModelApp" xmlns:fluent="clr-namespace:MoralesLarios.FluentMVVM.Infrastructure;assembly=MoralesLarios.FluentMVVM" fluent:AutoViewModelClass.ViewModelClassName="NoRuleViewModel" mc:Ignorable="d" Title="WithoutRuleView" Height="359" Width="602"> <Grid> </Grid> </Window>
AS we can check it don't meets naming rule.
WithoutRuleViewModel.cs code.
namespace FluentMVVMExamplesDesc.AutoViewModelApp.ViewModels { using MoralesLarios.FluentMVVM; public class WithoutRuleViewModel : ViewModelBase { public WithoutRuleViewModel() { } } }
ViewModel
class automatically.
Result.
When we auto instantiate non IoC setup classes, these classes have to be parameterless constructor, otherwise, they will throw an exception
.
All process in video.
Example 4: ViewModel class without nomenclature rule with IoC
Same as in Example 2, to give more value to example, we will add a dependency injection in our
ViewModel
.
public class NoRuleViewModel : ViewModelBase { private readonly IMyInjection _myInjection; public NoRuleViewModel(IMyInjection myInjection) { _myInjection = myInjection; } }
In this moment will add our
WithRuleViewModel
and MyInjection
classes in IoC
configuration. With this action
public void RegisterTypes() { var builder = new ContainerBuilder(); builder.RegisterType<WithRuleViewModel>().AsSelf(); builder.RegisterType<NoRuleViewModel>().AsSelf(); builder.RegisterType<MyInjection>().As<IMyInjection>(); Container = builder.Build(); }
Result.
All process in video.
Download AutoViewModel (AutoViewModel proyect) Code
No hay comentarios :
Publicar un comentario